A step-by-step guide to setting up Google Play In-App Updates
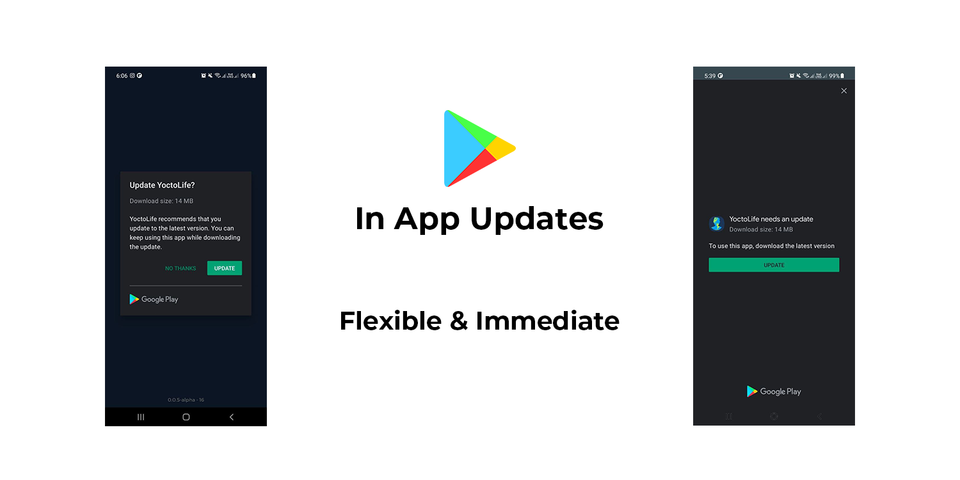
As an Android app developer, you may have found yourself wondering whether to "force" users to update your app or simply remind them to do so. Additionally, with a considerable number of users opting not to enable auto-updates or manually check for updates, it can become challenging to ensure they are using the latest version. Well, you can make your own implementation for "nicely asking" the users to update. Fortunately, the good news is that Google has already provided a solution for this, the Android In-App Updates feature.
Introduction
In-App Updates, as the name itself already self-explaining, is a functionality that enables updating the application from within the app itself. This convenient feature is available for devices running Android 5.0 (API 21) or later, but it requires the Play Core Library to be at least version 1.5.0. Additionally, for the feature to work seamlessly, the application must already be available on the Google Play Store. However, it's worth noting that this particular feature is not compatible with APK Expansion Files (.obb). Despite this limitation, it provides an effective way to keep your app's users up-to-date with the latest improvements and fixes.
In-App Updates offers two ways to carry out updates. The first method is called Flexible Updates, which comes in handy when you prefer a non-intrusive approach and the updates are minor in nature. With this option, users receive the choice to update the app in the background while continuing to use it, or they can opt not to update at that moment. On the other hand, the second method is known as Immediate Updates, which serves its purpose when you need to enforce updates, especially for critical fixes or essential improvements. When utilizing this method, users are immediately restricted from interacting with the application until they either update it or choose to close the application altogether. These two update options provide developers with the flexibility to accommodate different scenarios and user preferences efficiently.
How it works
After knowing Google Play In-App Updates support two ways updates. Maybe you wondering, how it actually works. Actually it's simple to make a flexible or immediate updates. First of all you can check if there is an update or not using AppUpdateManager, it will ask the Google Play if there is an update for your app using the package name of your app. Then, it will returning the update availability. If it's available you can start the update flow, either it's Flexible or Immediate update. That's it.
Well, if you want it to always using same flow over and over again then there is no other things that you need to do. For example, if you want your apps to be always using Flexible updates, then you didn't need to check whether it's need to start Flexible or Immediate updates. But what if you need? Then, you don't just need to start the flow. You also need to specify the flow based on your preferred way.
Google Play also give you other information along with the update availability. They give you Update Staleness and Update Priority, which will be useful if you want to have different flow for different cases.
The Update Staleness is an information about the number of days since the update became available on the Play Store. So if you want to decide to only Immediate update to the users that not updating after an update published for 1 month or so then you can use this.
The Update Priority is an information about how strongly to recommend an update to the user. It's ranging from 0 to 5, with 0 being the default and 5 being the highest priority. So if you want to decide to only Immediate update if the priority is the highest, which is 5. Then you can use this information.
But, unfortunately at this time of writing, Google Play Console doesn't have this kind of feature to edit or set the priority from the website. It's only available from Google Play Developer API.
How To Implement
After all of that information then I will give a step by step how to implement this feature on your apps, either its new app or existing app. The requirement here it's already uploaded to Google Play Store, not using APK Expansion Files (.obb), and can only be used on devices running Android 5.0 (API level 21) or higher.
Simple Example
The goal here on the simple example is to make an flexible or immediate updates without having logic for checking the Update Staleness and Update Priority. We will talk about that later on the next section of article, you can skip to the advanced example if you're more interested about that one.
OK First, we need to add the Play Core Library to the build.gradle (app). At this moment of writing the newest version is 1.10.0, make sure you're using the latest version. You can check the latest version here.
Then, in the Main activity we need to create an instance of the AppUpdateManager, request the update availability, then start the Flexible updates if there is update available. Here is the sample code.
That's it, you already added Google Play In-App Updates to your app using Flexible updates. You can simply change AppUpdateType.FLEXIBLE to AppUpdateType.IMMEDIATE to change to Immediate update flow.
Advanced Example
Now what if we need more than that? For example we want to use Update Staleness and Update Priority to make the decision whether we are going to use Immediate or Flexible updates. To make it simple, look at this table for our update strategy scenario.
PRIORTY | < 7 DAYS | > 7 DAYS |
---|---|---|
Priority 0 | Flexible | Flexible |
Priority 1 | Flexible | Flexible |
Priority 2 | Flexible | Flexible |
Priority 3 | Flexible | Immediate |
Priority 4 | Immediate | Immediate |
Priority 5 | Immediate | Immediate |
Based on that table, users with Priority 0 to 2 always receive Flexible Updates, regardless of update staleness. For Priority 4 and 5, users always get Immediate Updates. However, for Priority 3, the update type varies with update staleness. If the staleness is under 7 days, they receive Flexible Updates, but after 7 days, they receive Immediate Updates.
So we need to modify our code from the above one to cater this scenario. The changes that we need to do here are on the success listener of appUpdateInfoTask, instead of directly start the flow if the update available, we also need to check the staleness and priority. Here is the sample code:
Finished? Well, not really. We already handle the client side which will update it based on the priority and staleness but we aren't setting up the priority yet using Google Play Developer API.
Set Priority Using Google Play Developer API
To be able to upload to Google Play via the Play Developer API, we are required to link the Google Cloud project to the Google Play Console. This step is required if we want to use Update Priority in the In App Updates feature. If not, this section can be skipped and directly uploaded as usual using Google Play Console.
Linking Google Cloud Project to Google Play Console
- Open Google Play Console
- Choose Settings > Developer Account > API Access
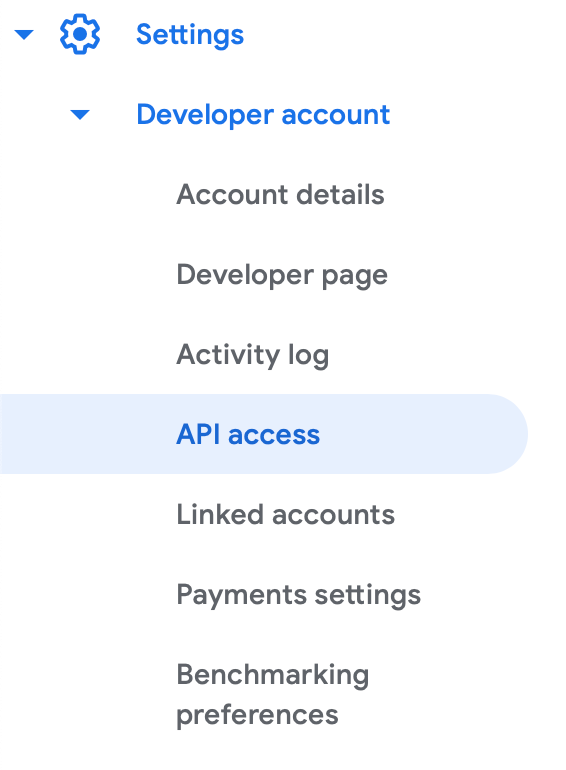
- Click Choose a project to link
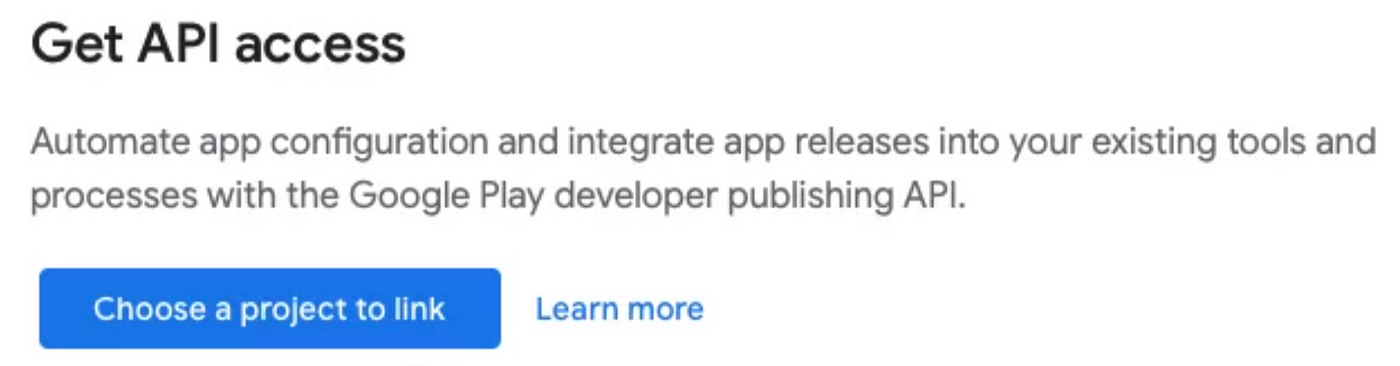
- If you already have a Google cloud project, choose Link existing project, if not, you can choose Create new project
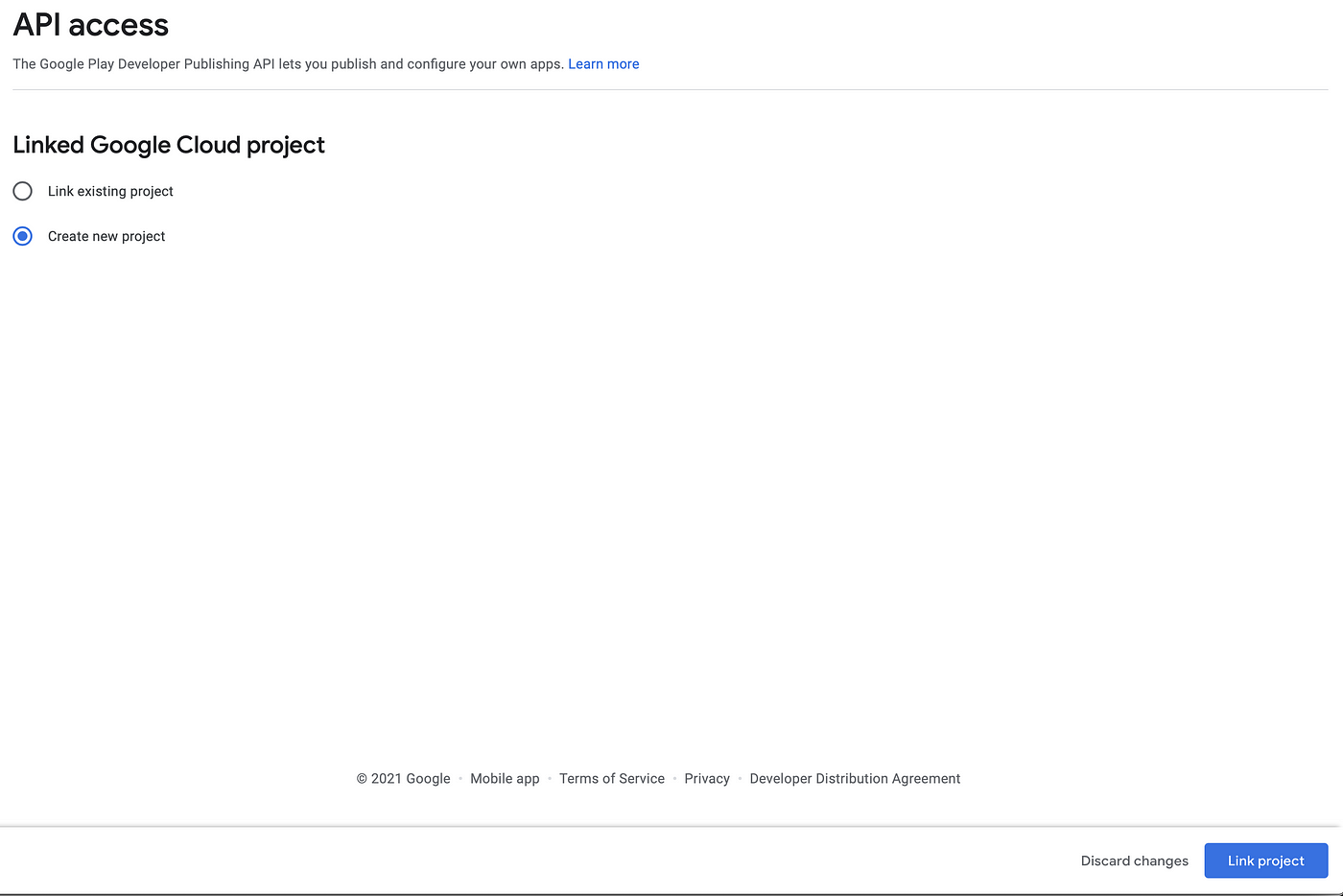
- Choose Create new service account

- A popup will appear like this, then open Google Cloud Platform
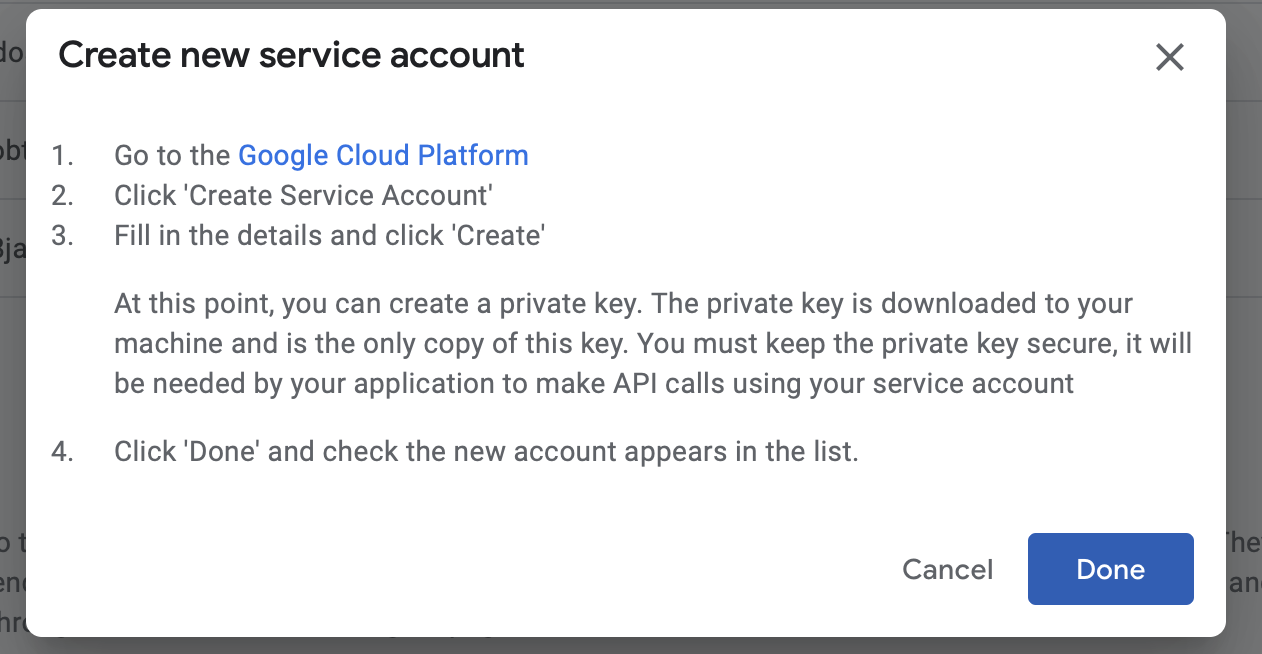
- Click on Create Service Account

- Fill in the name, and click Create
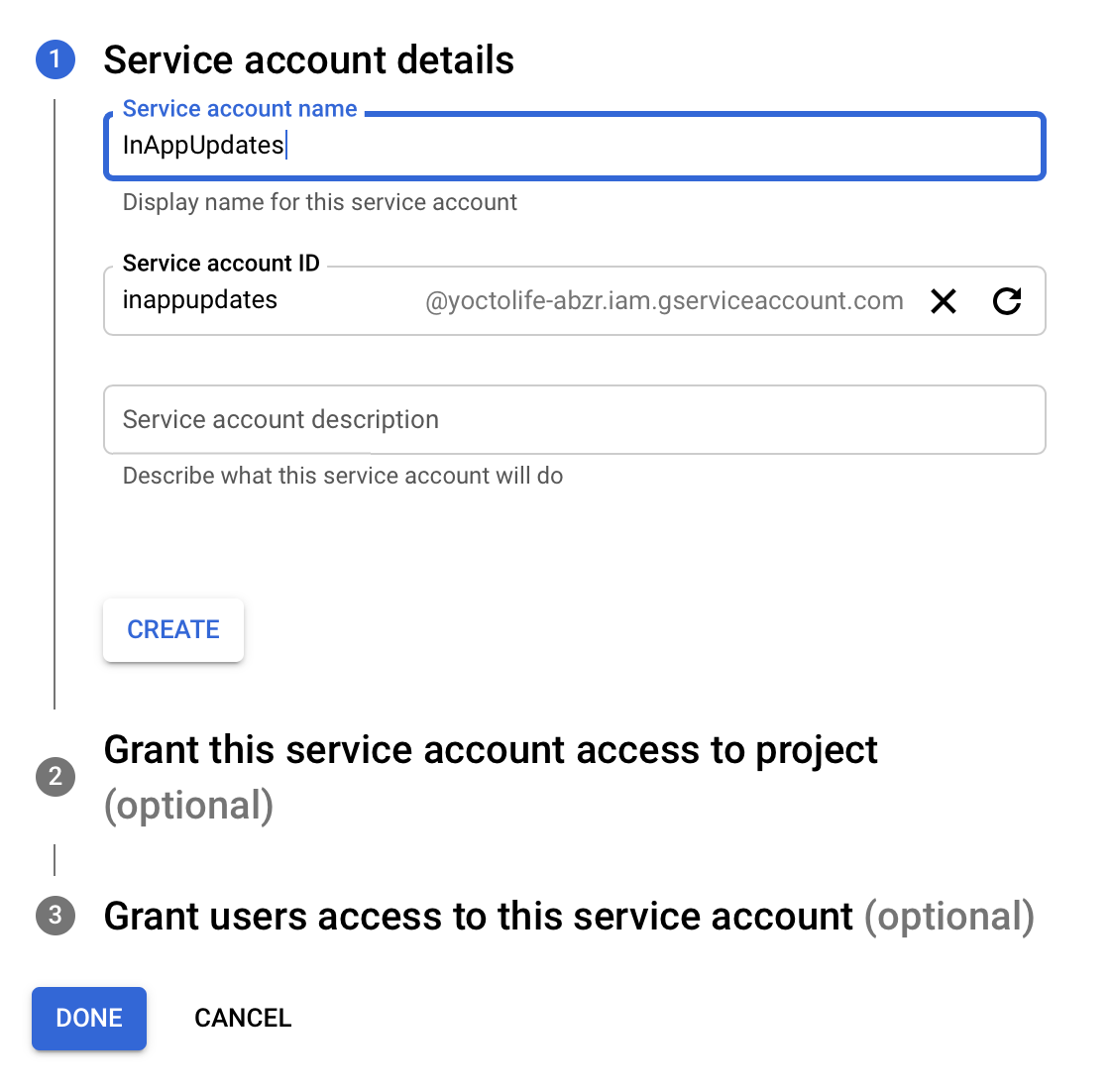
- In the next step, select Owner as the role, and click Done.
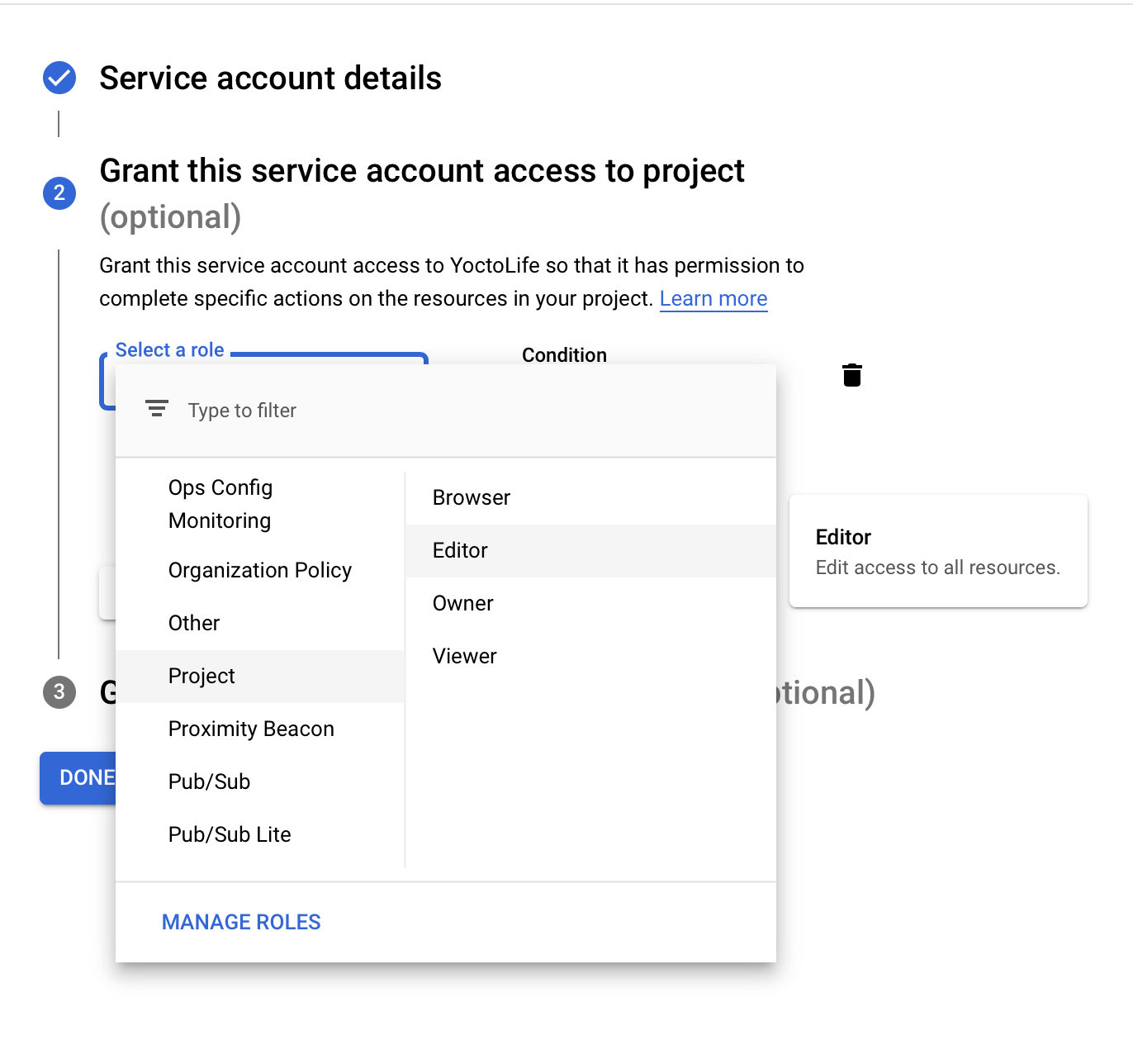
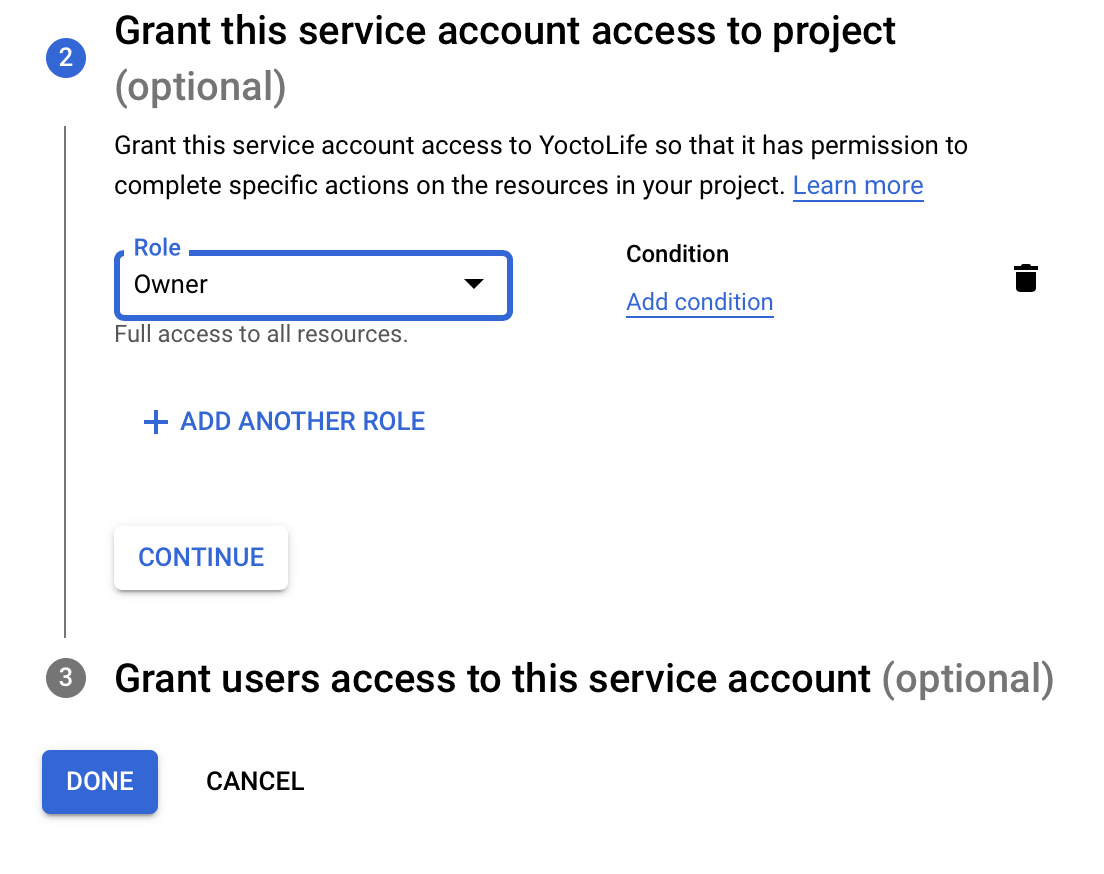
- Click the Actions icon and select Manage keys
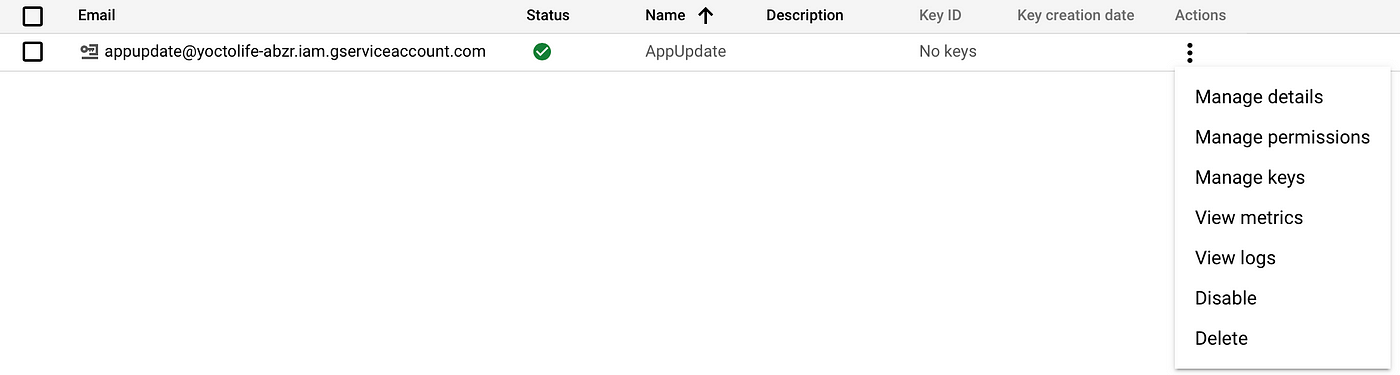
- Click Add Key dan choose Create new key
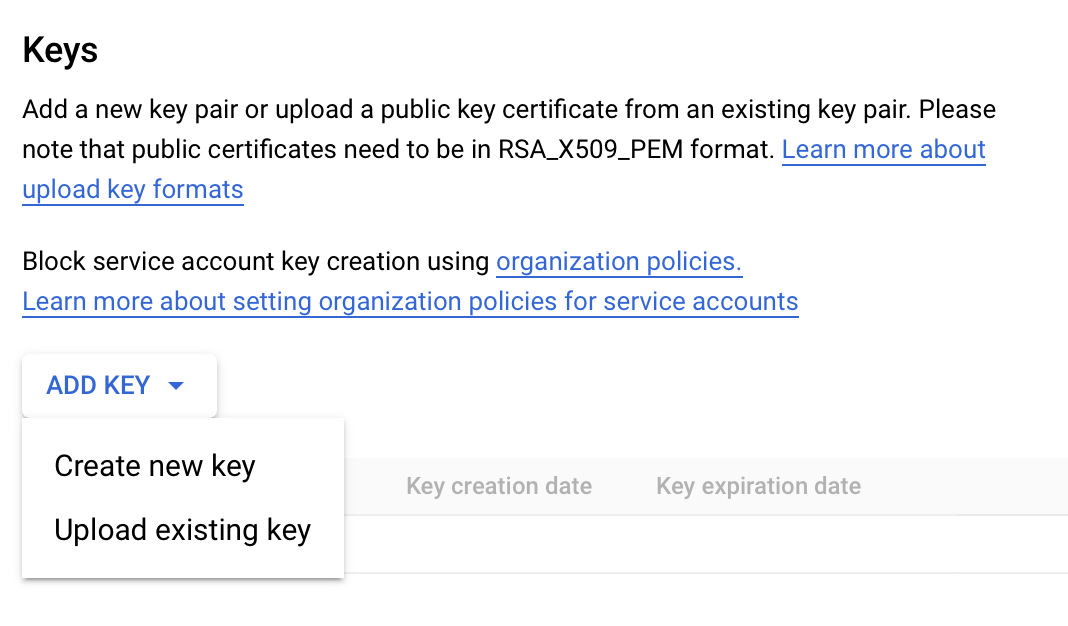
- Choose JSON and then click Create
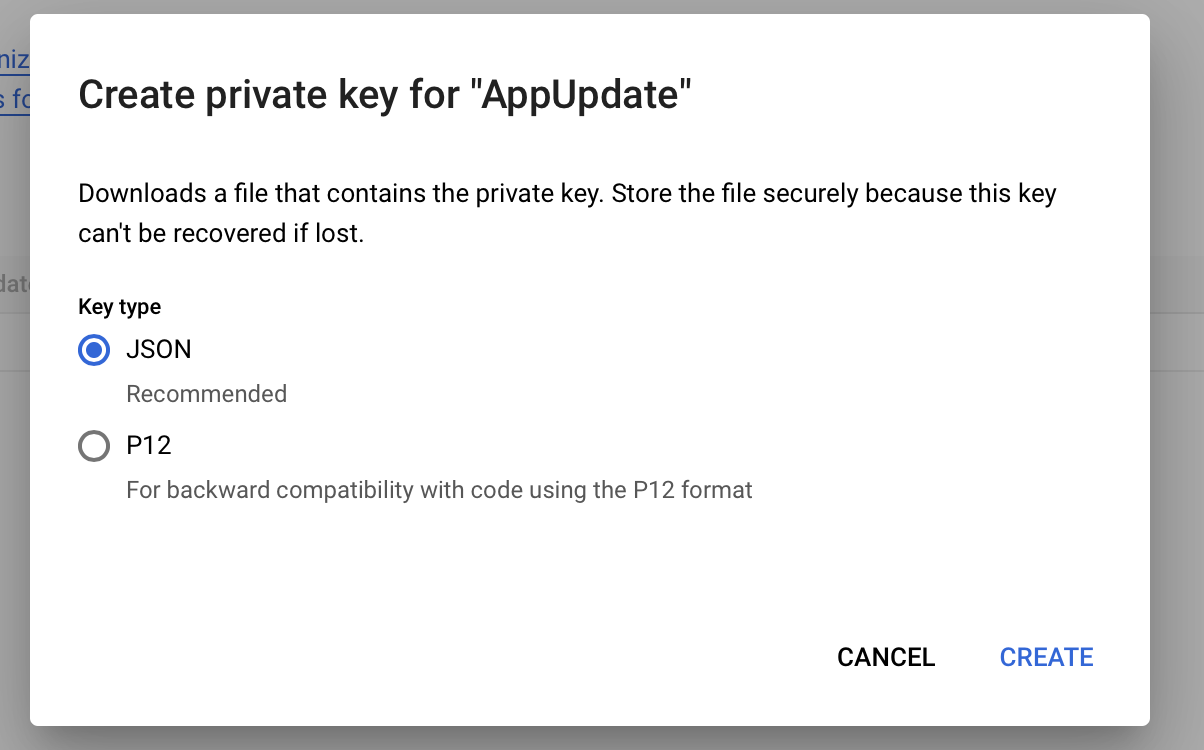
- Keep the downloaded file in a safe place, because it can't be re-downloaded if you lose it.
- Go back to Google Play Console, and click Done on this popup.
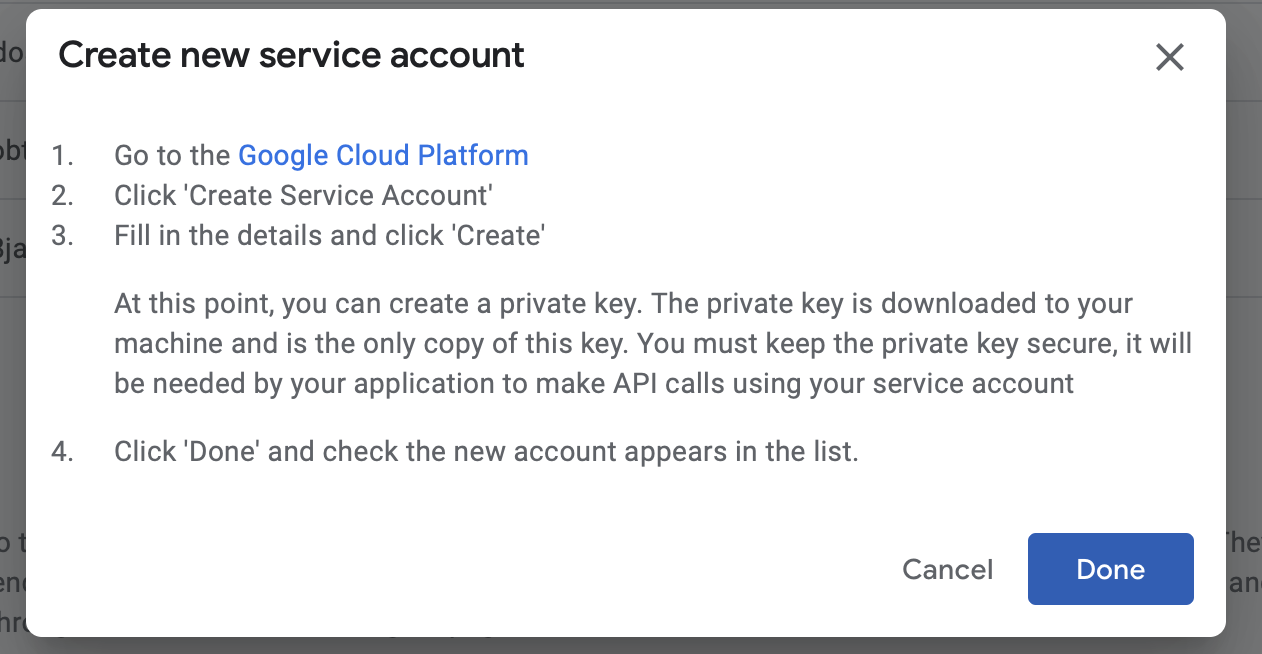
- Select Users and Permissions then Invite New User
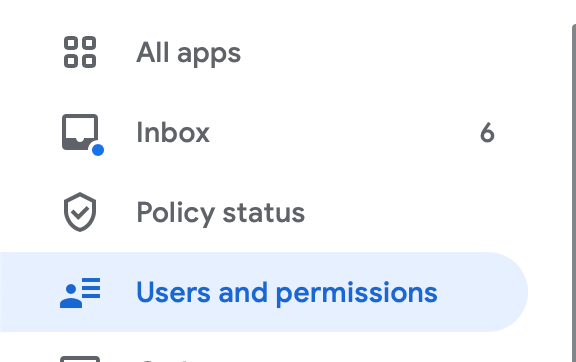
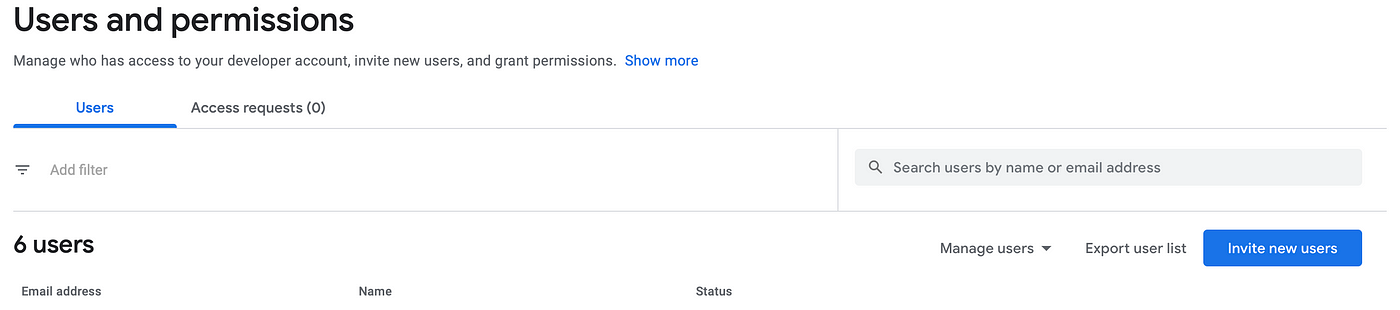
- Open the .json file, and copy client_email and paste it in Email Address, and click Add app at the bottom, and select the app you want to add In App Updates to.
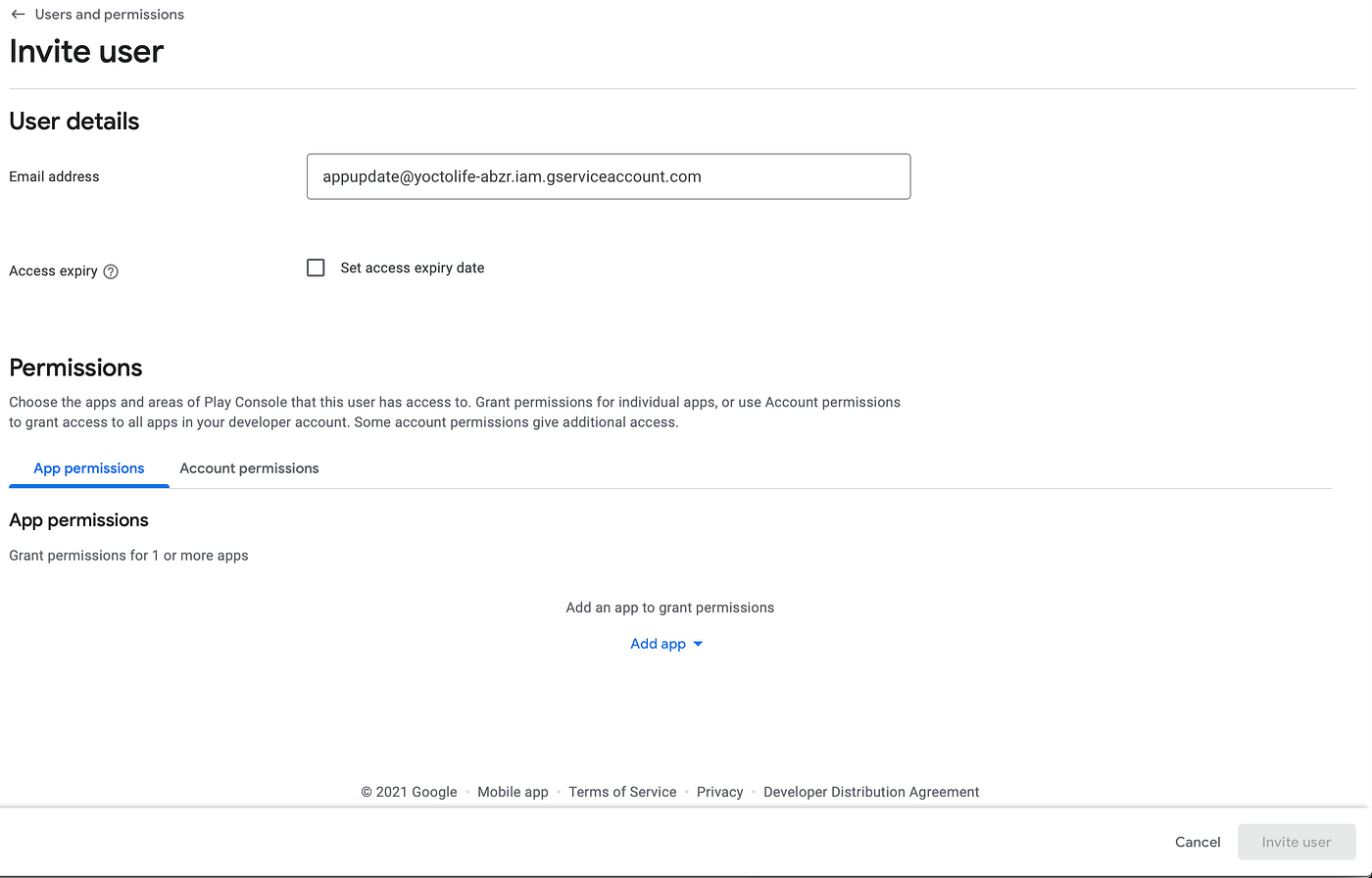
- In the permission popup, checklist 4 as shown below, and click Apply, and click Invite User.
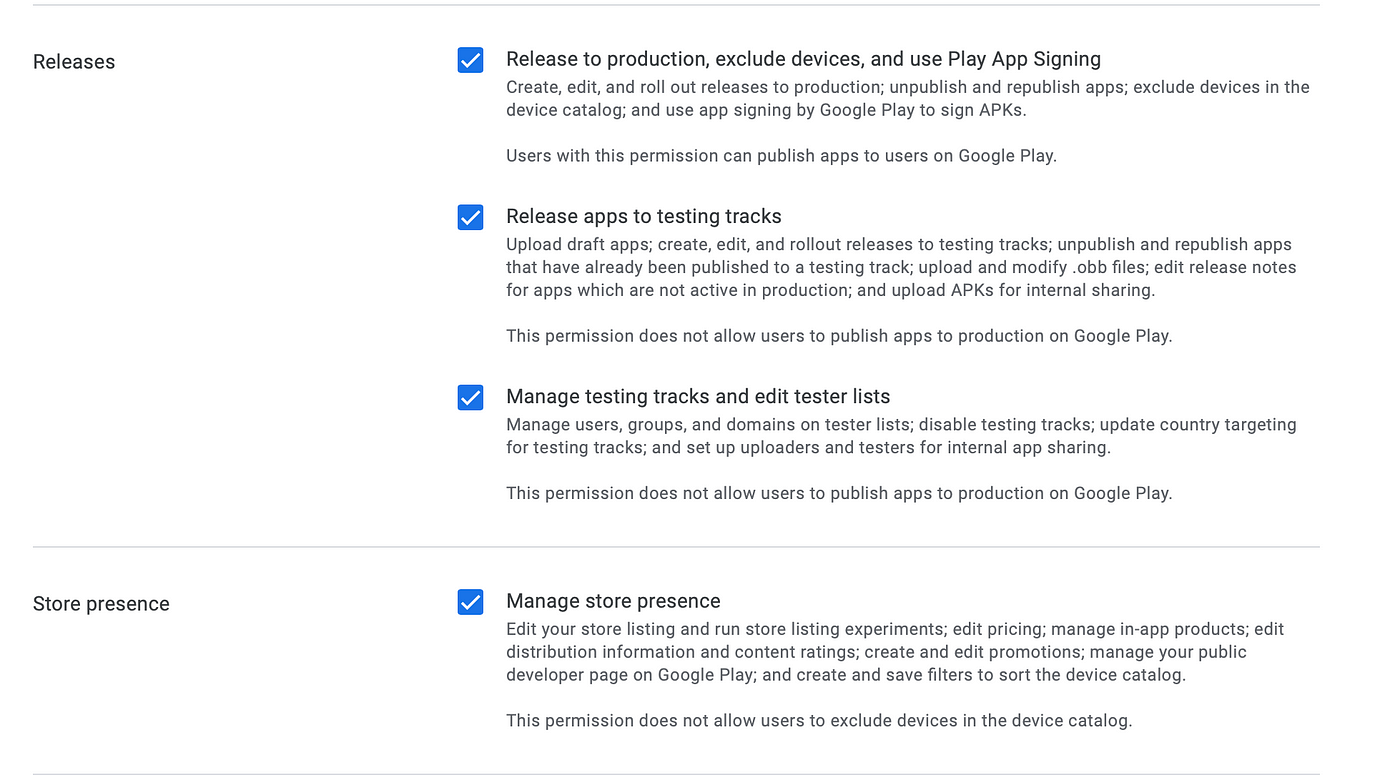
Now after doing all the steps above, we can upload the application via the Play Developer API to add Update Priority. Here we will use one of the packages that I made myself to facilitate the upload process called abizareyhan/playpublish. But before that, make sure the application containing the In App Updates code that has been written above has been uploaded to Google Play, you can use the normal method through the Google Play Console, without having to add Update Priority because the goal is for the In App Updates popup to appear, after that just proceed to the steps below.
Upload via Play Developer API
- First, install the playpublish package via npm
> npm i -g playpublish
- Run the playpublish command, and follow the remaining steps
> playpublish
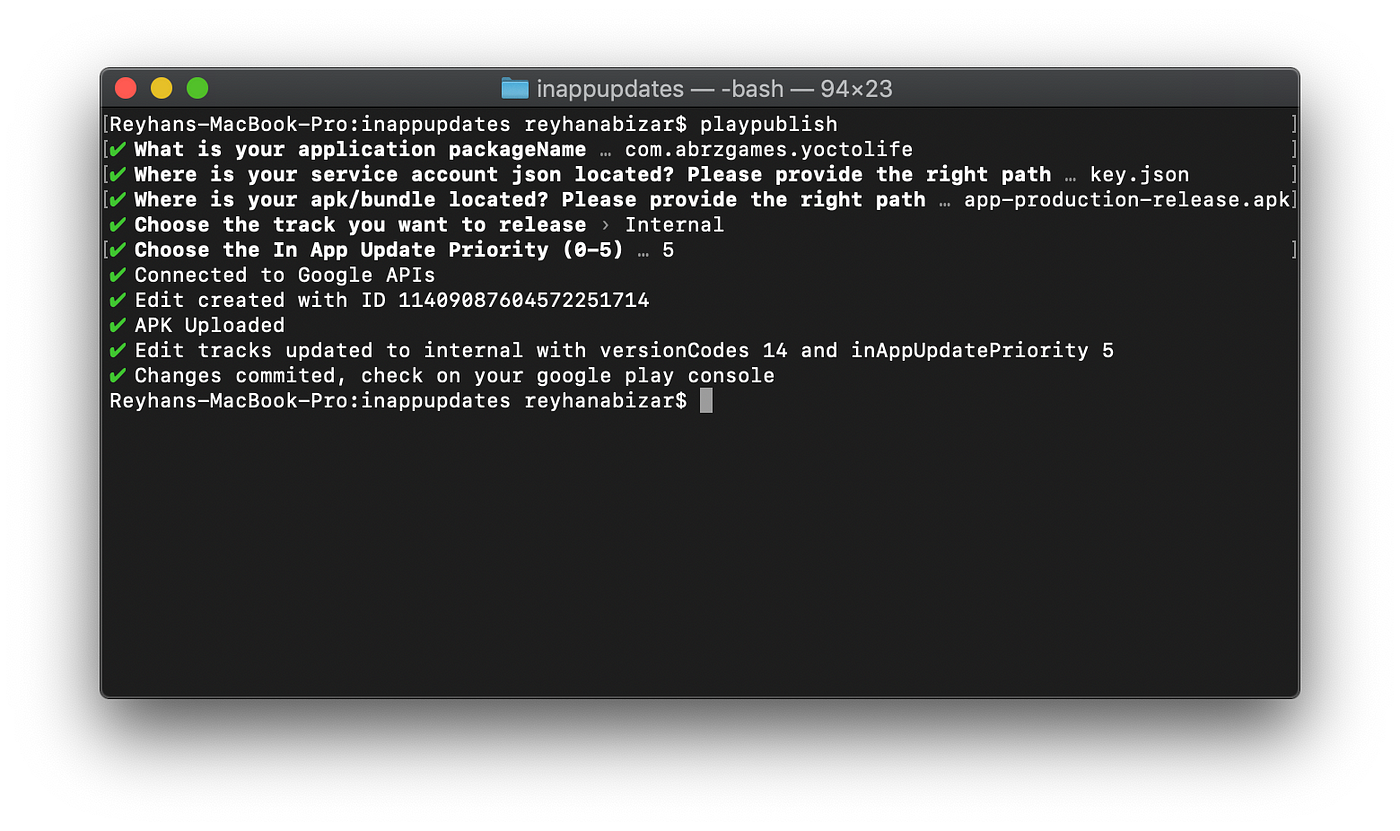
Try to keep the service account json and apk or app bundle files in the same folder to make it easier to write the path.
- If it works like the image above, you can check it directly in the Google Play Console. In this scenario what is uploaded is versionCodes 14 to the Internal Testing track and inAppUpdatePriority 5.
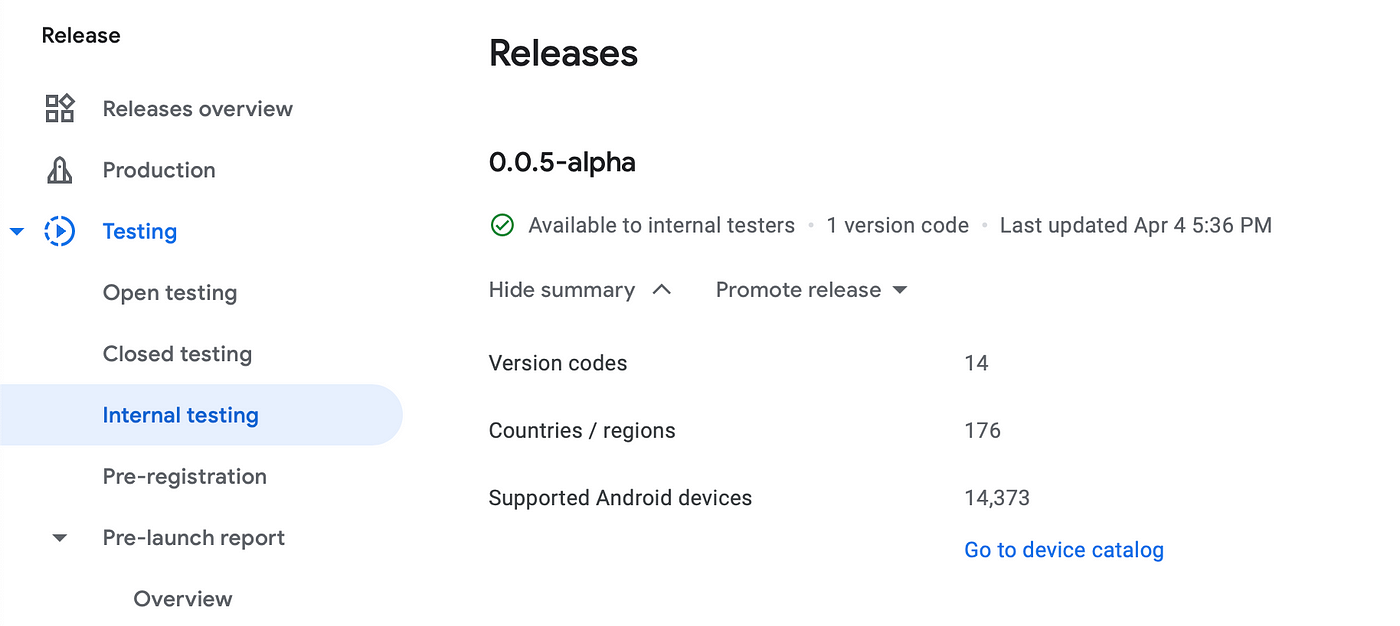
There you have it! Now you know all about In-App Updates and how to use them in your Android app. In-App Updates allow you to update your app from within the app itself, which makes it easier for users to stay up-to-date with the latest improvements and fixes.
So, with all this knowledge, you're ready to keep your app users happy and up-to-date with the latest features and bug fixes. Happy updating!